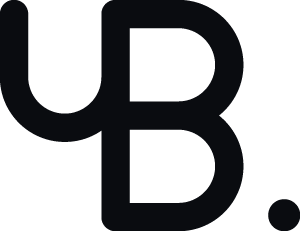
Hi,
and welcome! My name is Yuliia Batsheva. I am a software QA engineer from Ukraine, based in Lynnwood, Washington. I started off as a graphic designer, creating assets for web. Later on, I switched to more technical tasks, exploring coding, development and testing.
My preferred tools are JavaScript frameworks such as Webdriverio and Cypress for front-end test automation, Postman for API testing, Jira and TestRail as management and bug tracking systems. I continuously learn new tools and techniques.
My Recent Projects
RESTful API Testing
API request
POST {{baseURL}}/1/boards/?name={{boardName}}&defaultLists=false&key={{trelloKey}}&token={{trelloToken}}
pre-request script
let currentBoardNumber = pm.environment.get("currentBoardNumber"); if (currentBoardNumber) { currentBoardNumber++ } else { currentBoardNumber = 1; } let boardName = "My board " + currentBoardNumber; pm.environment.set("boardName", boardName); pm.environment.set("currentBoardNumber", currentBoardNumber);
test script
let response = pm.response.json(); pm.environment.set("boardID", response.id); pm.test("Status code is 200", function () { pm.response.to.have.status(200); }); pm.test("Board is created", function () { pm.expect(response.name).to.eql(pm.environment.get("boardName")); }) pm.test("Cookie exists", function() { pm.expect(pm.cookies.has("preAuthProps")).to.be.true; })
Trello API Testing with Postman
Creating collection of requests in Postman with implementation of collection environment, pre-request and test scripts. The collection follows basic user flow on Trello app.
As an example, I've added a request that creates a Trello board. It includes pre-request and test scripts that generate data with js (board name), parse the response and execute tests verifying that the board was created. Finally, the script sets collection variables that will be used for next requests. The variables are stored inside the environment and are used as query parameters and varibles for test scripts. The collection is automated, it can be run from CLI, and integrated with CI/CD tools.
Tools
Front-end Test Automation with WebdriverIO
Automating test framework for an e-commerce project. Here are examples of tests written in WebdriverIO using Mocha library.
The tests here belong to search functionality test suite and focus on the search box auto suggestions feature. These particular tests verify that:
- suggestion box is displayed when entering search query
- auto suggestions include the searched entry
- auto suggestions include links to the items
Finally, auto suggestions for a search entry are captured and
printed out to the console.
Page Object Model pattern was implemented so the selectors and
functions are defined and imported from search-page.js.
Tools
import searchPage from "../pages/search-page.js";
import searchData from "../data/searchData.js";
describe("Search functionality", () => {
beforeEach(async () => {
await browser.url("/");
});
it("should display relevant suggestions with links", async () => {
let searchEntry = searchData.searchEntry;
await searchPage.addSearchValue(searchEntry);
let suggestedItemLinks = await searchPage.suggestedLinks;
//auto suggestions box is displayed
await expect(suggestedItemLinks).toBeDisplayed();
//auto suggestions contain the searched word
for (let element of suggestedItemLinks) {
await expect(element).toHaveTextContaining(searchEntry);
}
//auto suggestions include links to the item
for (let element of suggestedItemLinks) {
await expect(element).toHaveLinkContaining(searchEntry);
}
//printing out suggested items
let suggestedItems = [];
for (let item of suggestedItemLinks) {
suggestedItems.push(await item.getText());
}
console.log(`Suggested itmes for ${searchEntry} are`,
suggestedItems.join(", "));
});
});
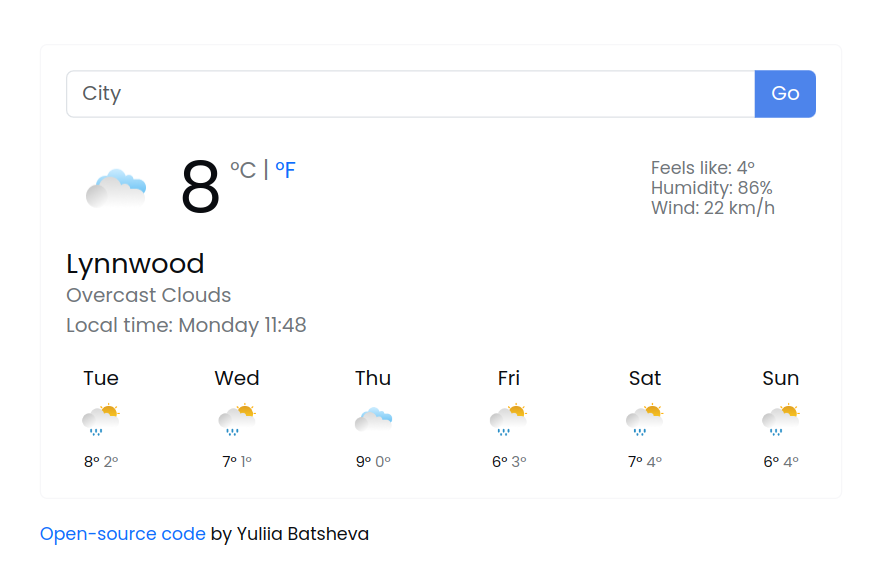
Vanilla JavaScript Weather App
Weather app created with HTML, CSS and JS. My practice project for front-end development and API integration. The app includes search functionality, units conversion option and provides weather forecast for next 6 days.
Tools